Exploring Llama.cpp With Practical Steps for Smarter AI Deployment
Published on Jun 13, 2025
Get Started
Fast, scalable, pay-per-token APIs for the top frontier models like DeepSeek V3 and Llama 3.3. Fully OpenAI-compatible. Set up in minutes. Scale forever.
Imagine wanting to run a high-quality language model for your next AI project, only to find out it is so big it won't fit on your machine. Then, you’re forced to either run the model in the cloud (with all the privacy and security risks that entails) or invest in expensive GPUs and complex infrastructure to run it locally. Fortunately, with recent developments in open-source AI, it’s now possible to run powerful language models locally, on your laptop, without breaking a sweat. In this post, we’ll explore how Llama CPP can help you achieve exactly that—run powerful, high-quality language models locally, without relying on the cloud, expensive GPUs, or complex infrastructure so that you can build smarter, faster, and more private AI applications.
One tool that facilitates achieving these objectives is Inference’s AI inference APIs. These can help you run powerful language models locally, without the need for complex infrastructure, to get you up and running quickly.
What is Llama CPP Framework and Its Key Features?
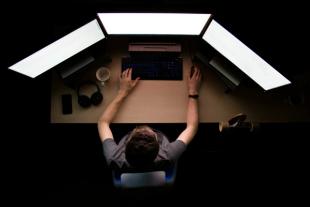
Llama.cpp is a lightweight C++ implementation of Meta’s LLaMA models, optimized for local inference without heavy dependencies. The purpose of Llama.cpp is to run LLMs efficiently on consumer hardware, especially CPUs. Key features include:
- CPU/GPU support
- Quantization
- Platform portability (macOS, Linux, Windows)
- Bindings for Python and other languages
Use cases include local chatbots, research, and edge AI applications.
Llama.cpp: The Specs
Llama.cpp is a large language model developed by Georgi Gerganov in 2023. It is an open-source library with a simple web interface. This Large Language Model is written in C/C++ with no dependencies. Llama.cpp is a popular open-source library hosted on GitHub with over 60,000 reviews, over 2,000 releases, and over 700+ developers.
Llama.cpp Simplifies Building and Deploying Advanced Applications
The primary objective of Llama.cpp is to provide a framework that allows for the efficient deployment of LLMs. It makes advanced applications more accessible and usable across various platforms with limited computational resources.
Llama.cpp Architecture: A Peek Under the Hood
Llama.cpp’s backbone is the original Llama models, which are also based on the transformer architecture. The authors of Llama leverage various improvements that were subsequently proposed and use different models, such as PaLM. The main difference between the LLaMa architecture and the transformers is:
- Pre-normalization (GPT3): Used to improve the training stability by normalizing the input of each transformer sub-layer using the RMSNorm approach instead of normalizing the output.
- SwigGLU activation function (PaLM): The original non-linearity ReLU activation function is replaced by the SwiGLU activation function, which leads to performance improvements.
- Rotary embeddings (GPTNeao): The rotary positional embeddings (RoPE) were added at each layer of the network after removing the absolute positional embeddings.
Features of Llama.cpp Framework
Check some of the significant features highlighted in the Llama framework mentioned below:
Lightweight Model
The complete Llama.cpp framework is written in pure C++ for efficiency. It involves multiple dependencies, which makes it easy to compile and run on various cross-platforms. It can also run without requiring specialized hardware, such as graphics processing units (GPUs).
Highly Portable
The complete platform is portable and can run on various platforms without any external dependencies.
Multi-Platform Support
This AI framework can run on macOS, Windows, iOS, and Android applications. It works on x86, ARM, and other architectures. It supports Raspberry Pi, and edge devices for low-power inferences.
Quantization Support
Llama.cpp supports GGML (General Graph Machine Learning)-based quantization techniques. It reduces model size significantly while maintaining reasonable accuracy. This LLM allows LLaMA models to run on devices with limited RAM (e.g., 8GB machines).
Efficient CPU & GPU Inferences
The Llama framework is optimized for CPU inference, making it usable on regular desktops and laptops. It also supports GPU acceleration via CUDA, Metal, OpenCL, and Vulkan for better performance.
Multi-threaded Performance Optimization
It utilizes efficient multi-threading to accelerate inference on CPUs, with optimized memory handling for running large models within limited hardware resources.
Easy Integration
Llama supports LLaMA 2 and other GGML-based models (e.g., Mistral, Alpaca, Vicuna). It can be integrated with Python, Rust, and other programming languages and is compatible with:
- Ollama
- Hugging Face
- Private LLM models
Working of Llama.cpp Model
Llama.cpp is an efficient and lightweight framework designed to run Meta’s LLaMA models on local devices, such as:
- CPUs
- GPUs
But have you ever wondered how these models work in real life? Let us go through the complete working of the Llama framework.
Model Loading
When a user loads a model in the Llama, the C++ framework reads GGML format model files from the disk, which are often quantized, thereby reducing memory consumption while maintaining accuracy. Llama.cpp is optimized to run on CPUs using advanced memory management and parallel processing.
The framework initializes all necessary parameters, including weights, biases, and attention mechanisms, to prepare the model for inference.
Tokenization for Input Processing
Before generating responses, Llama.cpp tokenizes the input text using Byte Pair Encoding (BPE) or a similar tokenization algorithm. Tokenization breaks the input text into smaller tokens (words or subwords), which the model understands. Each token is mapped to a corresponding numerical representation, allowing the model to process it efficiently.
Model Inference with Text Generation
Once the tokens are processed, Llama.cpp runs the transformer model’s forward pass to generate the following probable tokens. The self-attention mechanism enables the model to understand contextual relationships between words, ensuring coherent and contextually relevant responses.
Post Processing with Output Generation
After inference, the generated tokens are converted back into human-readable text using de-tokenization. The output is then formatted and displayed to the user. Llama.cpp supports streaming outputs, meaning text is displayed progressively rather than waiting for the entire response to be generated.
Optimisation for Effective Performance
Llama.cpp is optimized for running large models on limited hardware through several techniques. The quantization in Llama.cpp significantly reduces memory usage, allowing 7B, 13B, and even 65B models to run on consumer hardware. The framework also supports GPU acceleration via:
- CUDA (NVIDIA)
- Metal (Apple)
- OpenCL
- Vulkan
This further improves inference speed.
Related Reading
How Llama.cpp Works
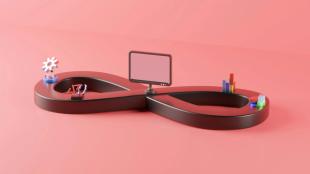
At its heart, llama.cpp is a lightweight, CPU-optimized inference engine built in C++ that enables the use of Meta’s LLaMA language models entirely offline, with low resource requirements. It focuses on:
- Quantization to reduce model size
- Memory-mapped inference for efficiency
- Multithreading to utilize all CPU cores
Model Quantization (Compression)
Large models like LLaMA 2 are gigabytes in size when using full-precision floats (FP16/FP32). To make them usable on machines with limited RAM or no GPU, llama.cpp supports quantized models in GGUF format. These quantized models reduce memory usage and computation by using 4-bit, 5-bit, or 8-bit integers, e.g.:
- Q4_0, Q5_1, Q8_0 — different levels of quantization
- Smaller size means faster load time and lower RAM footprint
Memory Mapping with Mmap
llama.cpp uses memory mapping (mmap) to load models efficiently. Instead of loading the whole model into RAM, it streams only the parts needed at any moment. This:
- Minimizes memory usage
- Speeds up inference
- Makes large models possible on modest hardware
Tokenization and Inference
Here’s what happens when we send a prompt:
- Tokenization: The text prompt is broken into tokens using LLaMA’s tokenizer.
- Feed forward: These tokens are passed through the neural network layers (transformers).
- Sampling: The model samples the next token using parameters like temperature, top_p, and stop.
- Decoding: Tokens are converted back to human-readable text.
This loop continues until the desired number of tokens is reached or a stop condition is met.
CPU Multithreading
llama.cpp uses multithreading to parallelize computations across multiple CPU cores. We can configure the number of threads with: llm = Llama(model_path="...", n_threads=8) This allows faster generation, especially on modern multi-core CPUs. Now that we know how llama.cpp works, let’s learn how we can install llama.cpp on our local machine in the next section.
How to Install llama.cpp Locally
Before we install llama.cpp locally, let’s have a look at the prerequisites:
- Python (Download from the official website)
- Anaconda Distribution (Download from the official website)
After downloading and installing the prerequisites, we start the llama.cpp installation process.
Step 1: Create a virtual environment
A virtual environment is an isolated workspace within our system where we can install and manage Python packages independently of other projects and the system-wide Python installation. This is particularly helpful while working on multiple Python projects that may require different versions of packages or dependencies.
To create a virtual environment on the local machine, run this command in the terminal:
- conda create--name vir-env
Conda is an open-source environment management system primarily used for managing Python and R programming language environments. It comes bundled with the Anaconda distribution. In the command, we’ve used conda create to create a virtual environment named vir-env, specified by the--name flag.
Step 2: Activate the virtual environment
Activate the newly created virtual environment vir-env using the conda activate command:
- conda activate vir-env
Step 3: Install the llama-cpp-python package
The llama-cpp-python package is a Python binding for LLaMA models. Installing this package will help us run LLaMA models locally using llama.cpp. Let’s install the llama-cpp-python package on our local machine using pip, a package installer that comes bundled with Python:
- pip install llama-cpp-python
Next, let’s discuss the step-by-step process of creating a llama.cpp project on the local machine.
Setup and Installation of Llama Cpp: On macOS & Linux
- Windows Setup
- Choosing the Right Binary
If you’re downloading pre-built binaries from Llama.cpp’s releases page [Link], choose based on your CPU and GPU capabilities:
- AVX (llama-bin-win-avx-x64.zip): For older CPUs with AVX support.
- AVX2 (llama-bin-win-avx2-x64.zip): For Intel Haswell (2013) and later.
- AVX-512 (llama-bin-win-avx512-x64.zip): For Intel Skylake-X and newer.
- CUDA (llama-bin-win-cuda-cu11.7-x64.zip): If using an NVIDIA GPU.
Ensuring CUDA Compatibility for GPU Acceleration
If unsure, start with AVX2 as most modern CPUs support it. For GPUs, ensure that your CUDA driver version matches the binary version of the CUDA toolkit. For this tutorial, I have CUDA 12.4 installed on my PC, so I downloaded the llama-b4676-bin-win-cuda-cu12.4-x64.zip and cudart-llama-bin-win-cu12.4-x64.zip and unzipped them, placed the binaries in a directory, and added this directory to my path environment variables.
Linux & macOS Setup
For Linux and macOS, download the appropriate binaries:
- Linux: llama-bin-ubuntu-x64.zip
- macOS (Intel): llama-bin-macos-x64.zip
- macOS (Apple Silicon M1/M2): llama-bin-macos-arm64.zip
After downloading, extract the files and add the directory to your system’s PATH to execute commands globally. You can also use the following installation using curl in Linux:
- curl -fsSL https://ollama.com/install.sh | sh
After downloading the right files, unzipping and adding the extracted directory to your system’s environment variables to run the executables from any location, we are now ready to explore the functionalities of llama.cpp.
Understanding GGUF, GGML, Hugging Face, and LoRA Formats
GGUF (Generalized GGML Unified Format) is an optimized file format designed for running large language models efficiently using Llama.cpp and other frameworks. It improves compatibility and performance by standardizing how model weights and metadata are stored, enabling efficient inference across different hardware architectures.
What is GGML?
GGML (Generalized Gradient Model Language) is an earlier format used for LLM inference that supports quantized models, making them more memory-efficient. Nevertheless, GGUF has largely replaced GGML due to its enhanced features and improved performance.
Converting GGML to GGUF
Suppose you have a GGML model and need to use it with Llama.cpp, you can convert it to GGUF using a conversion script.
Example command
Python convert_llama_ggml_to_gguf.py - input model.ggml - output model.gguf
The convert_llama_ggml_to_gguf.py script exists in the llama.cpp github repository in the leading directory.
Hugging Face Format
Hugging Face models are typically stored in PyTorch (.bin or .safetensors) format. These models can be converted into GGUF format using conversion scripts, such as convert_hf_to_gguf.py. Text generation model with the huggingface format `.safetensors`
LoRA Format
LoRA (Low-Rank Adaptation) is a fine-tuning technique used to adapt large language models to specific tasks efficiently. LoRA adapters store only the fine-tuned weight differences rather than modifying the entire model. To use LoRA with Llama.cpp, you may need to merge LoRA weights with a base model before conversion to GGUF using convert_lora_to_gguf.py.
Downloading GGUF Model Files from Hugging Face
You can download GGUF model files from Hugging Face and use them with Llama.cpp. Follow these steps:
- Visit Hugging Face Models Page: Go to Hugging Face and search for LLaMA or any model compatible with GGUF. In this tutorial, we will use the mistral gguf files downloaded from this link.
- Download the Model: Navigate to the model’s repository and download the GGUF version of the model. If the GGUF format is not available, you may need to convert it manually as explained before.
- Move the File: Place the downloaded or converted GGUF model into your models/ directory.
Downloading the GGUF Model File for the Llama-3.2–1B-instruct Model
- Run a model
- Now we can use the llama-cli command, which is one of the executables we downloaded.
- You can check all the flags that can be used with the llama-cli command to trigger the LLM model using the gguf file.
At the end of the help list of the llama-cli utility, there are two examples of triggering a text generation and a chat:
- Interacting with the Mistral-7B instruct model using the GGUF file and llama-cli utility from llama.cpp
- Interacting with Llama.cpp in Python
Overview of llama-cpp-python
The llama-cpp-python package provides Python bindings for Llama.cpp, allowing users to:
- Load and run LLaMA models within Python applications.
- Perform text generation tasks using GGUF models.
- Customize inference parameters like temperature, top-k, and top-p for more controlled responses.
- Run models efficiently on both CPU and GPU (if CUDA is enabled).
- Host models as an API server for easy integration into applications.
Installing Required Packages
You can use llama-cpp-python, which provides Python bindings for llama.cpp:
- Pip install llama-cpp-python
- Running Inference in Python
- Now we can use the llm model gguf file that we have downloaded above, load it in python using the llama_cpp package, and trigger the chat completion function.
From llama_cpp import Llama
llm = Llama(model_path="mistral-7b-instruct-v0.2.Q2_K.gguf")
response = llm.create_chat_completion(
messages=[
{
"role": "user",
"content": "how big is the sky"
}
])
print(response)
The response will be something like:
plaintext
{
'id': 'chatcmpl-e8879677-7335-464a-803b-30a15d68c015',
'object': 'chat.completion',
'created': 1739218403,
'model': 'mistral-7b-instruct-v0.2.Q2_K.gguf',
'choices': [
{
'index': 0,
'message':
{
'role': 'assistant',
'content': '
The size of the sky is not something that can be measured in a way that is meaningful to us, as it is not a physical object with defined dimensions. The sky is the expanse of space above the Earth, encompassing both the atmosphere and the outer space beyond. It goes on forever in all directions, as far as our current understanding of the universe extends.
So, we cannot assign a specific size to the sky. Instead, we can describe the size of particular parts of the universe, such as the diameter of a star or the distance between two galaxies.'
},
'logprobs': None,
'finish_reason': 'stop'
}
],
'usage': {
'prompt_tokens': 13,
'completion_tokens': 112,
'total_tokens': 125
}
}
Downloading and Using GGUF Models with Llama.from_pretrained
The Llama.from_pretrained method allows users to directly download GGUF models from Hugging Face and use them without manually downloading the files.
Example
From llama_cpp import Llama
Download and load a GGUF model directly from Hugging Face
llm = Llama.from_pretrained(
repo_id="TheBloke/Mistral-7B-Instruct-v0.2-GGUF",
filename="mistral-7b-instruct-v0.2.Q4_K_M.gguf"
)
response = llm.create_chat_completion(
messages=[
{"role": "user", "content": "How does a black hole work?"}
]
)
print(response)
Simplified Model Management
This method simplifies the process by automatically downloading and loading the required model into memory, eliminating the need to place GGUF files in a directory manually and load the GGUF file from that directory.
'id': 'chatcmpl-e8879677-7335-464a-803b-30a15d68c015',
'object': 'chat.completion',
'created': 1739218403,
'model': 'mistral-7b-instruct-v0.2.Q2_K.gguf',
'choices': [
{
'index': 0,
'message':
{
'role': 'assistant',
'content': '
The Immeasurable Expanse of the Sky
The size of the sky is not something that can be measured in a way that is meaningful to us, as it is not a physical object with defined dimensions. The sky is the expanse of space above the Earth, encompassing both the atmosphere and the outer space beyond. It goes on forever in all directions, as far as our current understanding of the universe extends.
So, we cannot assign a specific size to the sky. Instead, we can describe the size of particular parts of the universe, such as the diameter of a star or the distance between two galaxies.'
},
'logprobs': None,
'finish_reason': 'stop'
}
],
'usage': {
'prompt_tokens': 13,
'completion_tokens': 112,
'total_tokens': 125
}
}
You can use the cache_dir parameter to specify the directory where the model will be downloaded and cached.
Running Llama.cpp as a Server
You can run llama.cpp as a server and interact with it via API calls.
Start the Server
llama-server -m mistral-7b-instruct-v0.2.Q2_K.gguf
Launching the model as a server in your terminal will give the following response:
- Send Requests Using Python
- Import requests
Define the API endpoint
url = "http://localhost:8000/completion"
Define the payload
payload = {
"model": "mistral-7b-instruct-v0.2.Q4_K_M.gguf",
"prompt": "How big is the sky?",
"temperature": 0.7,
"max_tokens": 50
}
headers = {"Content-Type": "application/json"}
Try response = requests.post(url, json=payload, headers=headers)
Check if the request was successful. If response.status_code == 200:
Parse the response JSON
- response_data = response.json()
Extract the result from the response
choices = response_data.get("choices", [])
if choices:
result = choices[0].get("text", "")
print("Response:", result)
else:
print("No choices found in the response.")
else:
print(f"Request failed with status code {response.status_code}: {response.text}")
except Exception as e:
print(f"Error occurred: {e}")
The Immeasurable Expanse of the Sky
The response will be something like: The sky is not a tangible object and does not have physical dimensions, so it cannot be measured or quantified in the same way that we measure and quantify objects with size or dimensions.
Send Requests from Terminal (Linux/macOS) or PowerShell (Windows)
curl -X POST "http://localhost:8000/completion" \
-H "Content-Type: application/json" \
-d '{"prompt": "Tell me a fun fact.", "max_tokens": 50}'
Related Reading
Common Errors While Creating a llama.cpp Project
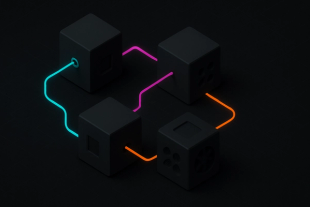
Missing Dependencies: The Most Common Hurdle in Llama CPP Projects
When you try to build a Llama CPP project and get an error that looks something like this:
Could not find a package configuration file provided by "ggml" (requested version "0.0.0").
The following CMake variables may aid in locating ggml: ggml_DIR (variable) ... Config file ggml-config.cmake was not found.
/Config file ggml-config-version.cmake was not found.
This error typically indicates that you're missing dependencies required to build the project. In this case, the `ggml` dependency is missing. The best way to fix this is to install the necessary dependencies with the following commands:
bash
sudo apt install cmake build-essential python3
Unsupported Compiler Versions: Make Sure You Have the Right One
If you see errors that mention something about "C++ standards" or "modern C++," you are probably using an old version of GCC or Clang that doesn't support the version of C++ that Llama CPP needs to build. To fix this, install a newer version of the compiler. You will want at least GCC 10 or Clang 11. You can check your version by running the following command:
bash g++ --version
File Not Found—Check Your Paths when Loading Models
An error like this:
- Error: file not found: ggml-model.bin
Troubleshooting Model Loading Errors
Usually, it means there's a problem with loading a model that your project is trying to access. To fix this, check the file path for typos. File paths are case-sensitive, so ensure the model file is named exactly as your code expects. Also, verify that the model has been downloaded and is in the correct format (.gguf).
Out-of-Memory Errors: Reduce Your Context Size
If you get a segmentation fault or memory allocation failure when running a Llama CPP project, you are likely running out of memory. To fix this, try reducing the context size in your code and using smaller models.
Start Building with $10 in Free API Credits Today!
LLM inference is the process of using a trained LLM to generate predictions on new data. This process is similar to how other machine learning models work. First, an LLM is trained on a large dataset (such as the Internet) until it can create reliable outputs. Afterward, the model can be used to generate predictions based on new input.
In the case of LLMs, these predictions can include generating text outputs, classifying and tagging inputs, and even creating embeddings for seamless integration into vector databases.
The Benefits of Running LLM Inference in the Cloud
LLM inference can be run locally or in the cloud. Running inference in the cloud is often superior to local results. For one, cloud inference results can be accessed from anywhere, allowing for better collaboration across teams and organizations.
Enhanced Security and Scalability Through Cloud LLM Inference
Using cloud capabilities means that businesses don’t have to worry about local hardware limitations that can slow down results or even prevent LLM inference from being run altogether. Finally, cloud inference can be more secure, as user data can be processed and stored on secure cloud servers without needing to be retained locally.